Link: github.com/edu-bm7/Philosophers
As part of my journey as a Software Engineer, I undertook the challenge of solving the Dining Philosophers Problem. This classic synchronization problem involves finding a solution to allow a group of philosophers to dine peacefully, while adhering to certain constraints.
In this project, I faced two distinct sets of requirements for solving the Dining Philosophers Problem, each with its own unique constraints and approach.
First Solution: Threads and Mutex
In the first solution, I had to represent each philosopher as a separate thread. The constraints were that philosophers couldn't communicate with each other, and two forks were required for a philosopher to eat. To achieve this, I utilized mutexes (mutual exclusion) to ensure exclusive access to forks by the philosophers. A single thread acted as an Arbiter, monitoring the philosophers' activities and determining when the simulation should terminate.
Second Solution: Processes and Semaphores
The second solution posed different challenges. Here, I represented each philosopher as a separate process, but with the constraint that the main process could not be a philosopher. Philosophers still couldn't communicate with each other, and the forks were placed in the center of the table without any shared memory or global variables allowed to be used and with forks having no state in memory. Instead, I employed semaphores to represent the availability of forks. An interesting aspect of this solution was that I couldn't use signals for inter-process communication (IPC). To overcome this limitation, I introduced an Arbiter Thread for each process, using the kill() function as a means of IPC to signal when a philosopher had completed its task, facilitating the termination of the simulation.
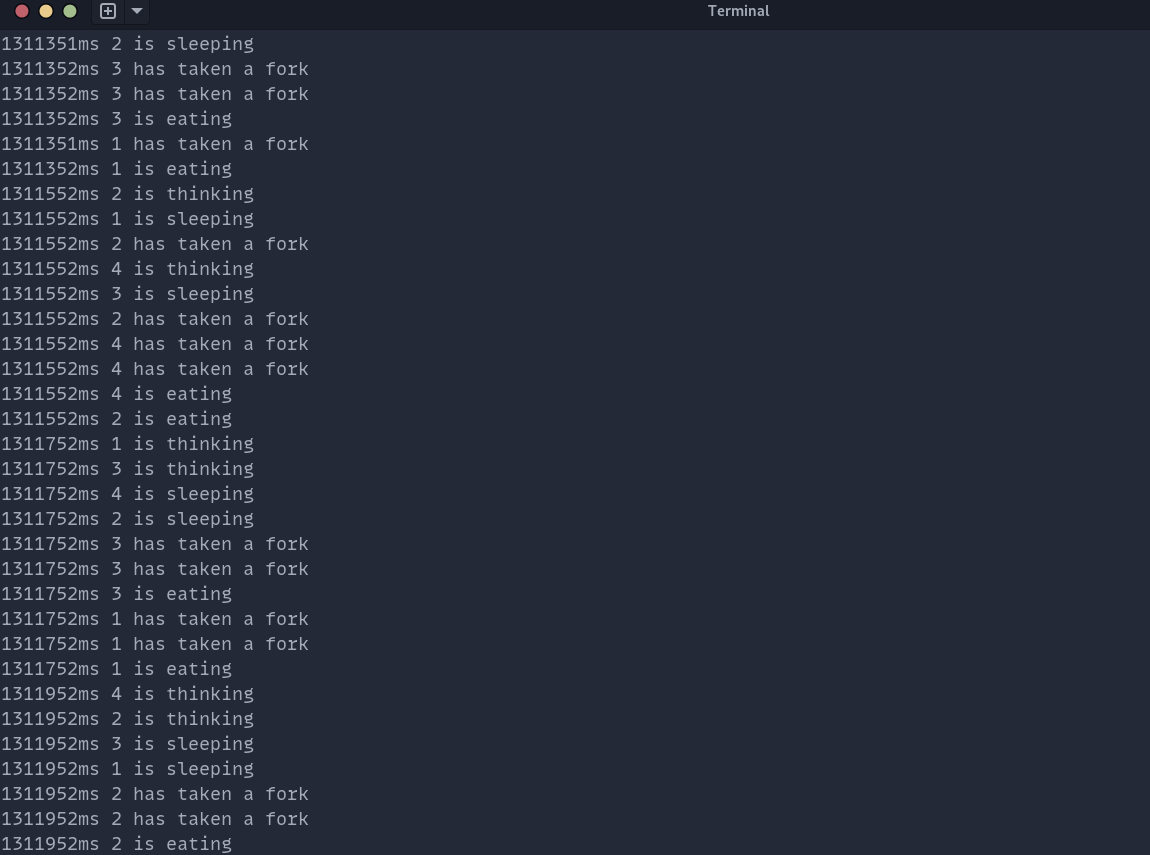